Interactions
The default behavior of ACRA is to send crash reports silently. From the application user point of view, the app closes, and that's all. Though, a report has been sent without the user being aware of it.
Depending on the state of your application and your concern of your users data plan usage and private data handling, you might prefer notifying them that a crash report has been sent, or even ask them the authorization to send one... and why not ask them to describe what they were doing during the crash. The following section details those user interactions.
Silent
Nothing shown, nothing to configure.
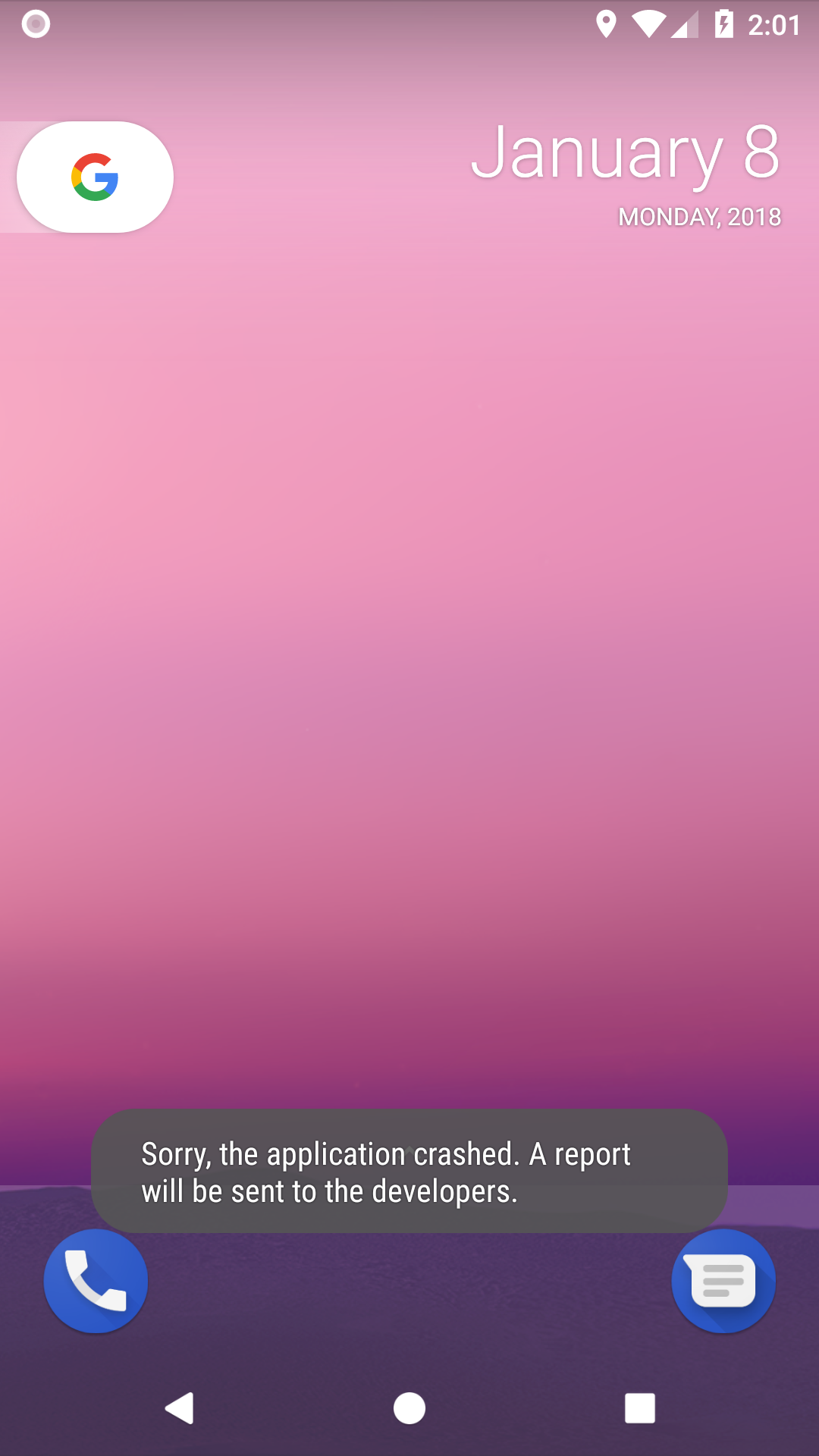
Toast
A Toast with your text is shown. No user interaction.
- Kotlin
- Java
toast {
//required
text = getString(R.string.toast_text)
//defaults to Toast.LENGTH_LONG
length = Toast.LENGTH_LONG
}
new ToastConfigurationBuilder()
//required
.withText(getString(R.string.toast_text))
//defaults to Toast.LENGTH_LONG
.withLength(Toast.LENGTH_LONG)
.build()
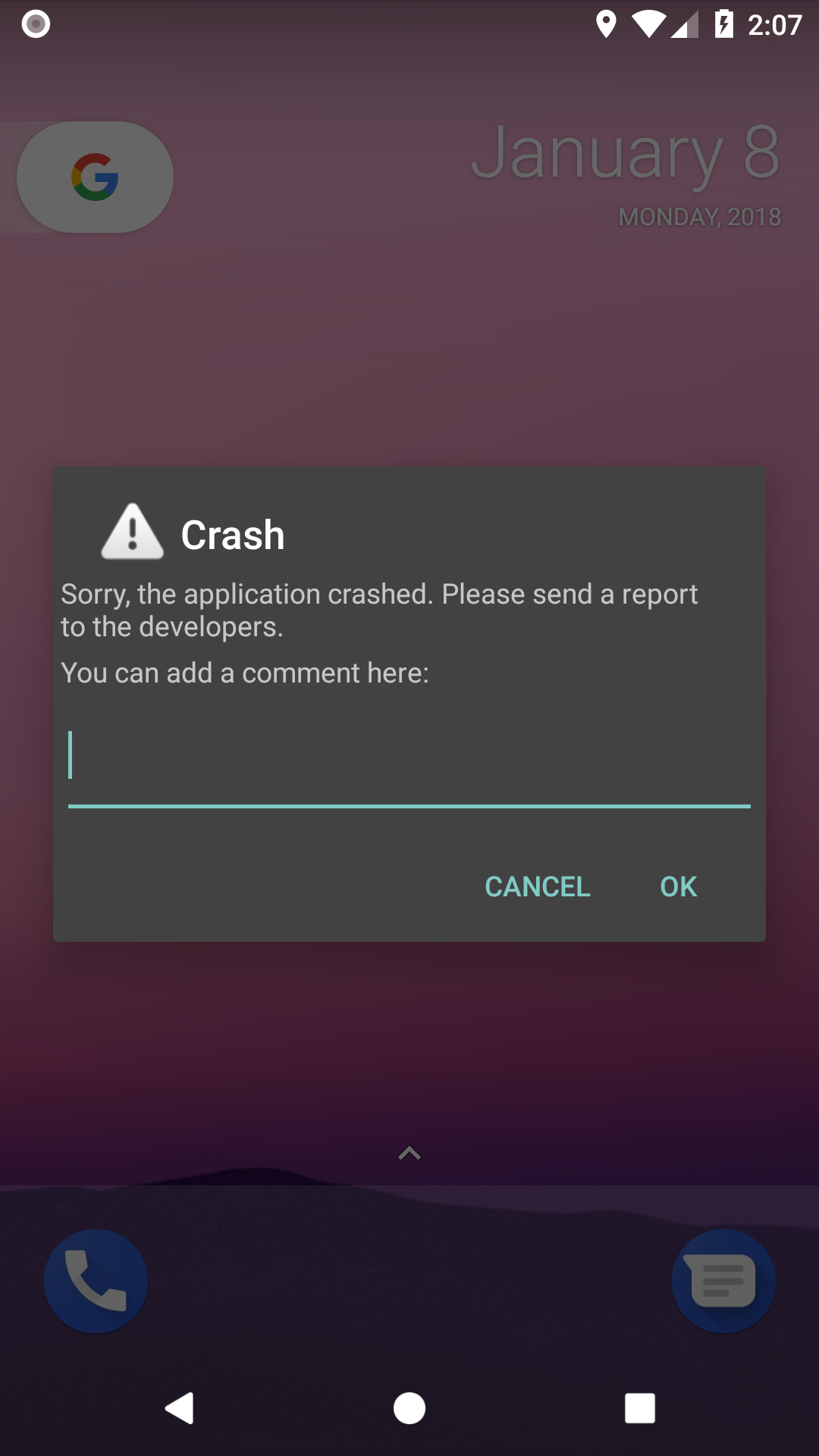
Dialog
A widely customizable dialog is shown. A report will only be sent if the user agrees.
- Kotlin
- Java
dialog {
//required
text = getString(R.string.dialog_text)
//optional, enables the dialog title
title = getString(R.string.dialog_title)
//defaults to android.R.string.ok
positiveButtonText = getString(R.string.dialog_positive)
//defaults to android.R.string.cancel
negativeButtonText = getString(R.string.dialog_negative)
//optional, enables the comment input
commentPrompt = getString(R.string.dialog_comment)
//optional, enables the email input
emailPrompt = getString(R.string.dialog_email)
//defaults to android.R.drawable.ic_dialog_alert
resIcon = R.drawable.dialog_icon
//optional, defaults to @android:style/Theme.Dialog
resTheme = R.style.dialog_theme
//allows other customization
reportDialogClass = MyCustomDialog::class.java
}
new DialogConfigurationBuilder()
//required
.withText(getString(R.string.dialog_text))
//optional, enables the dialog title
.withTitle(getString(R.string.dialog_title))
//defaults to android.R.string.ok
.withPositiveButtonText(getString(R.string.dialog_positive))
//defaults to android.R.string.cancel
.withNegativeButtonText(getString(R.string.dialog_negative))
//optional, enables the comment input
.withCommentPrompt(getString(R.string.dialog_comment))
//optional, enables the email input
.withEmailPrompt(getString(R.string.dialog_email))
//defaults to android.R.drawable.ic_dialog_alert
.withResIcon(R.drawable.dialog_icon)
//optional, defaults to @android:style/Theme.Dialog
.withResTheme(R.style.dialog_theme)
//allows other customization
.withReportDialogClass(MyCustomDialog.class)
.build()
If you need more control than the configuration provides, you can set your own dialog activity in reportDialogClass
. Remember, activities have to be registered in the AndroidManifest.xml
:
<activity
<!-- required -->
android:name="my.package.MyCustomDialog"
android:process=":acra"
<!-- recommended -->
android:excludeFromRecents="true"
android:finishOnTaskLaunch="true"
android:launchMode="singleInstance"/>
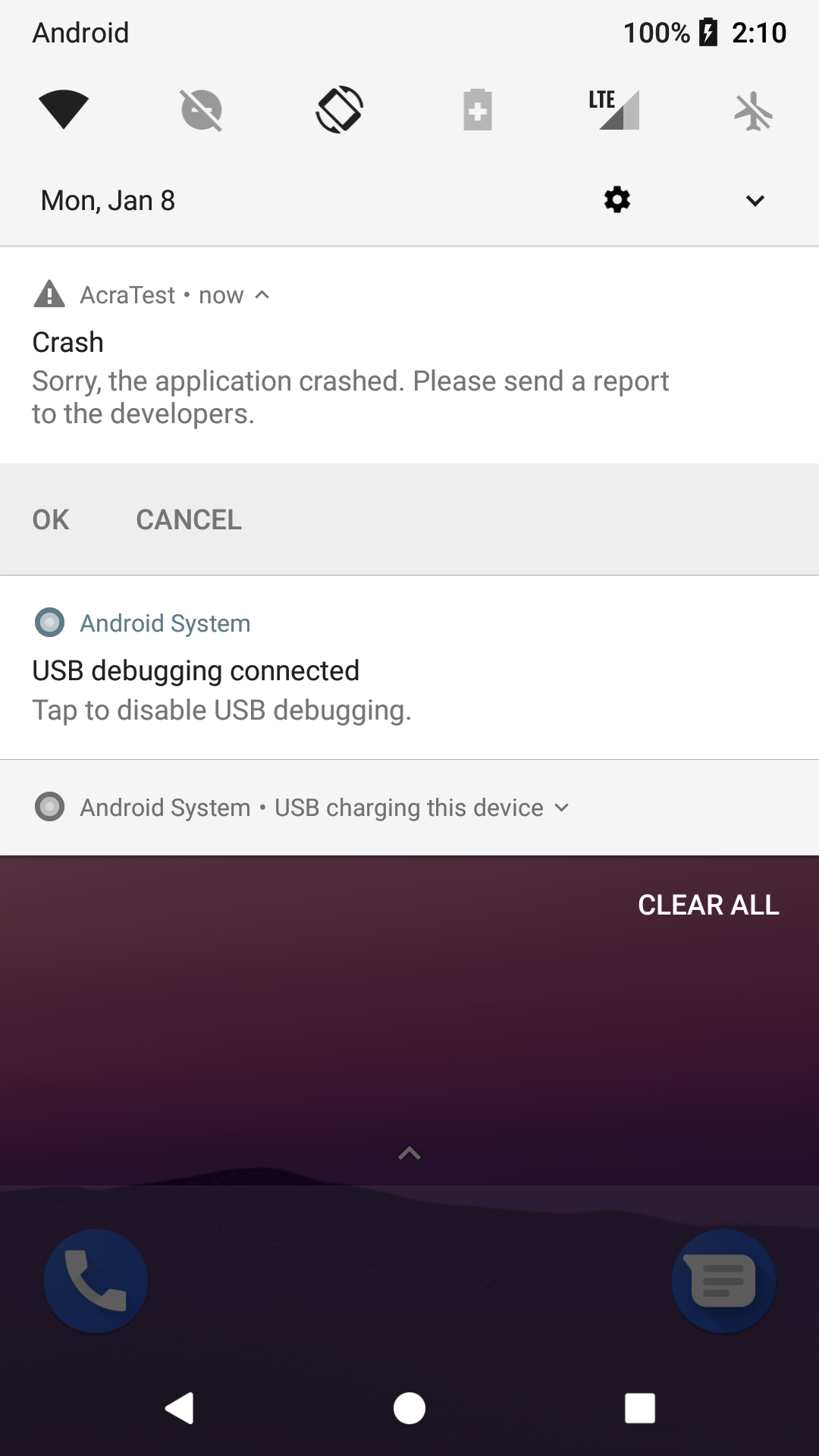
Notification
A notification is shown. A report will only be sent if the user agrees.
If the user has disabled this notification channel in the Android settings, no report will be sent! This may be the default setting. It is your own responsibility to ask the user to allow these notifications.
- Kotlin
- Java
notification {
//required
title = getString(R.string.notification_title)
//required
text = getString(R.string.notification_text)
//required
channelName = getString(R.string.notification_channel)
//optional channel description
channelDescription = getString(R.string.notification_channel_desc)
//defaults to NotificationManager.IMPORTANCE_HIGH
channelImportance = NotificationManager.IMPORTANCE_MAX
//optional, enables ticker text
tickerText = getString(R.string.notification_ticker)
//defaults to android.R.drawable.stat_sys_warning
resIcon = R.drawable.notification_icon
//defaults to android.R.string.ok
sendButtonText = getString(R.string.notification_send)
//defaults to android.R.drawable.ic_menu_send
resSendButtonIcon = R.drawable.notification_send
//defaults to android.R.string.cancel
discardButtonText = getString(R.string.notification_discard)
//defaults to android.R.drawable.ic_menu_delete
resDiscardButtonIcon = R.drawable.notification_discard
//optional, enables inline comment button
sendWithCommentButtonText = getString(R.string.notification_send_with_comment)
//required if above is set
resSendWithCommentButtonIcon = R.drawable.notification_send_with_comment
//optional inline comment hint
commentPrompt = getString(R.string.notification_comment)
//defaults to false
sendOnClick = false
}
new NotificationConfigurationBuilder()
//required
.withTitle(getString(R.string.notification_title))
//required
.withText(getString(R.string.notification_text))
//required
.withChannelName(getString(R.string.notification_channel))
//optional channel description
.withChannelDescription(getString(R.string.notification_channel_desc))
//defaults to NotificationManager.IMPORTANCE_HIGH
.withChannelImportance(NotificationManager.IMPORTANCE_MAX)
//optional, enables ticker text
.withTickerText(getString(R.string.notification_ticker))
//defaults to android.R.drawable.stat_sys_warning
.withResIcon(R.drawable.notification_icon)
//defaults to android.R.string.ok
.withSendButtonText(getString(R.string.notification_send))
//defaults to android.R.drawable.ic_menu_send
.withResSendButtonIcon(R.drawable.notification_send)
//defaults to android.R.string.cancel
.withDiscardButtonText(getString(R.string.notification_discard))
//defaults to android.R.drawable.ic_menu_delete
.withResDiscardButtonIcon(R.drawable.notification_discard)
//optional, enables inline comment button
.withSendWithCommentButtonText(getString(R.string.notification_send_with_comment))
//required if above is set
.withResSendWithCommentButtonIcon(R.drawable.notification_send_with_comment)
//optional inline comment hint
.withCommentPrompt(getString(R.string.notification_comment))
//defaults to false
.withSendOnClick(false)
.build()